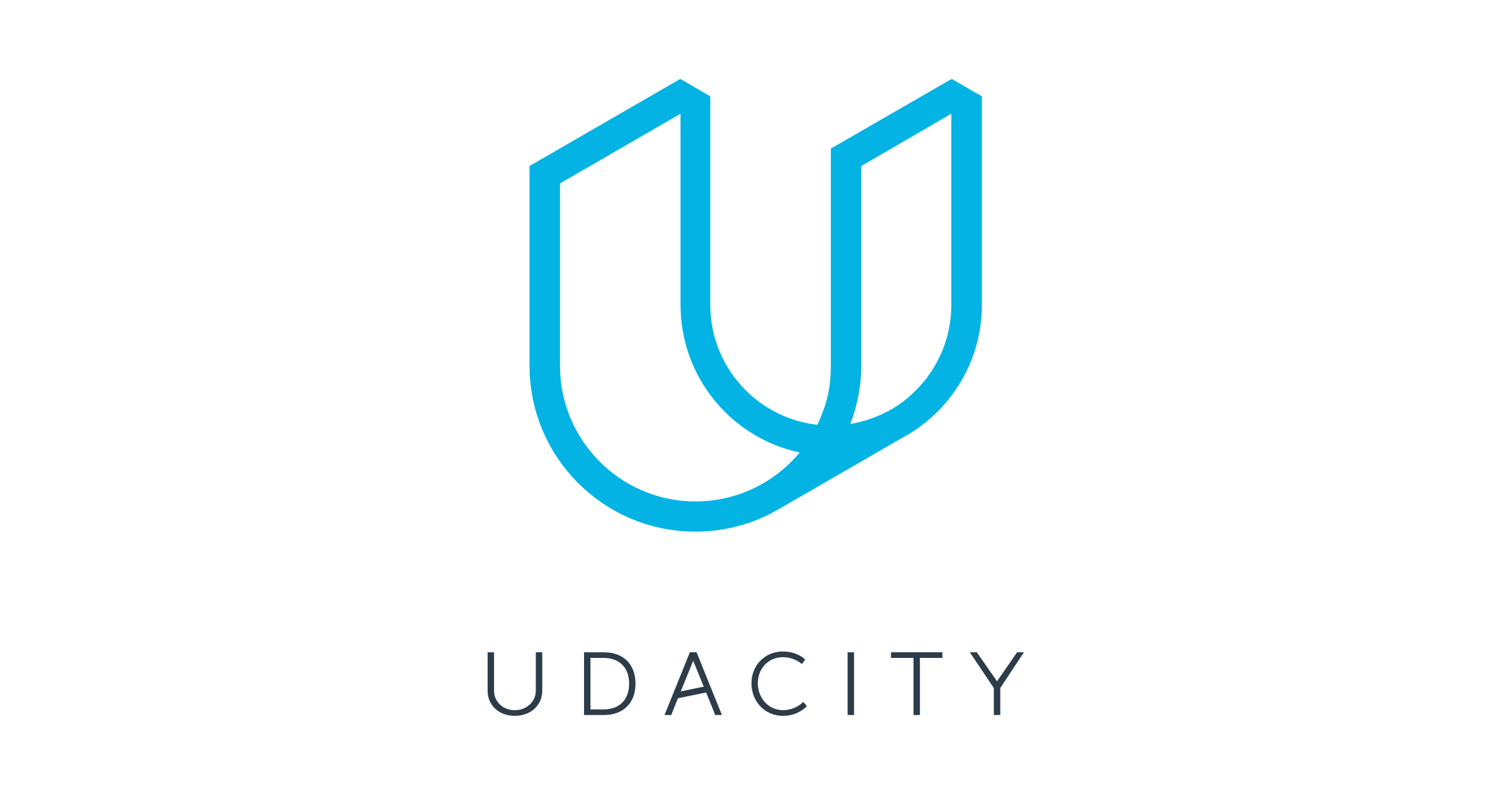
Experimento en curso de udacity que llevó a una libreria python.
La historia por detrás de la libreria Pythonbenchmark
Saltar la historia
La historia
Todo empezó en el curso de CS 101 en Udacity donde gracias a Un amigo que conocí en el foro me metí en un análisis interesante. Se trataba de dos algoritmos para hacer una tabla hash para un motor de búsqueda. El poder de los motores de búsqueda consiste en la velocidad con la cual logran asociar terminos de búsqueda con cientos de millones de posibles resultados. La arquitectura que utilizan motores de búsqueda como google es mucho mas compleja hablando de la totalidad pero se basan en algo bastante simple. Las tablas hash. Una tabla hash es una estructura de datos que asocia llaves o claves con valores. Hasta ahí todo normal, nada tan especial, el problema es que si existen millones de registros, el buscador tendría que correr a través de todo el registro cada vez que alguien haga una búsqueda, simplemente sería demasiado costoso y lento. Publicaré otro post explicando como se soluciona este problema, ahora siguiendo con el tema del curso. Según el profesor David Evans uno de los dos algoritmos que habíamos hecho en clases era más rápido, lo cual resultó no ser cierto como descubrió un amigo que abrió un POST en el foro de Udacity. Sólo le faltaba ayuda con el algoritmo de comparación. Esto capto inmediatamente mi interés y empece a dedicar mi sábado de noche a crear un algoritmo que tomaría nuestros dos algoritmos de la clase para comprobar cual de ellos es mas rápido.
Después de muchas pruebas llegamos a la conclusión que el profesor estaba equivocado, le avise por Twitter y me respondió en poco tiempo lo cual me sorprendió, profesores tan abiertos a críticas quisiera haber tenido más en la facultad.
@NiebuhrKarl @antoniogbo You are correct! Thanks for the notice - I'll post a longer discussion on this in the forum...
— David Evans (@UdacityDave) March 26, 2014
Siguió con un mail y un post explicando el motivo por el cual la teoría en este caso no acertó, por una cuestión de cómo esta implementado algo en python mismo. Recomiendo leer el foro para entenderlo mas a detalle. El post completo
Este fue el mail de Dave:
UdacityDave has just posted a new answer on Udacity Forums to the question Challenging the professor :) Testing times - need your help to analyze this fun discovery!:
Don't forget to come over and cast your vote. Thanks, P.S. You can always fine-tune which notifications you receive here.
|
La librería
Todo lo mencionado anteriormente pasó en 2014, un año mas tarde tuviendo una discusión en el chat con amigos programadores me puso a reutilizar ese código hecho para el curso para comparar dos algoritmos. El tema es el siguiente, timeit, el método que viene con la librería estándar de python permite medir el tiempo de ejecución de código esta diseñado para medir “code snippets”. (Snippet es un término del idioma inglés utilizado en programación para referirse a pequeñas partes recusables de código fuente.) Lo cual no me gusta para nada en la mayoría de casos, mayormente quiero comparar dos funciones y ver cuál es mas eficiente. No conociendo a ninguna librería que permite hacer eso me puse a crear mi propia librería https://github.com/Karlheinzniebuhr/pythonbenchmark/ .
En el documento readme están las indicaciones pero lo mencionaré aquí brevemente en español.
Básicamente hay dos formas de utilizarla, con el decorador, y llamando a la función compare() pasándo 2 funciones como parámetros.
Caso 1: Llamar utilizando el decorador
from pythonbenchmark import compare, measure
import time
a,b,c,d,e = 10,10,10,10,10
algo = [a,b,c,d,e]
def miFuncion(algo):
time.sleep(0.4)
def miFuncionOptimizada(algo):
time.sleep(0.2)
# test de comparación
compare(miFuncion, miFuncionOptimizada, 10, algo)
Caso 2: Utilizando la función compare
from pythonbenchmark import compare, measure
import time
a,b,c,d,e = 10,10,10,10,10
algo = [a,b,c,d,e]
# se declaran los decoradores encima de las dos funciones a comparar
@measure
def myFunction(algo):
time.sleep(0.4)
@measure
def myOptimizedFunction(algo):
time.sleep(0.2)
# se llama a las funciones para que se ejecuten
myFunction(algo)
myOptimizedFunction(algo)
Si bien este método no esta tan preciso como el método timeit que viene inlcuido con python, es lo suficientemente preciso para la mayoría de casos. La precisión en este caso esta definida por la granularidad del reloj de la plataforma (sistema operativo) en donde se esta ejecutando el programa. Espero que a más personas les sirva la librería, recibo sugerencias y contribuciones con gusto. Saludos
** UPDATE **
La libreria ahora esta disponible en el gestor de paquetes PyPi. Podes instalarla con este comando:
pip install pythonbenchmark
Comments